
Be a bounty hunter in my tavern.
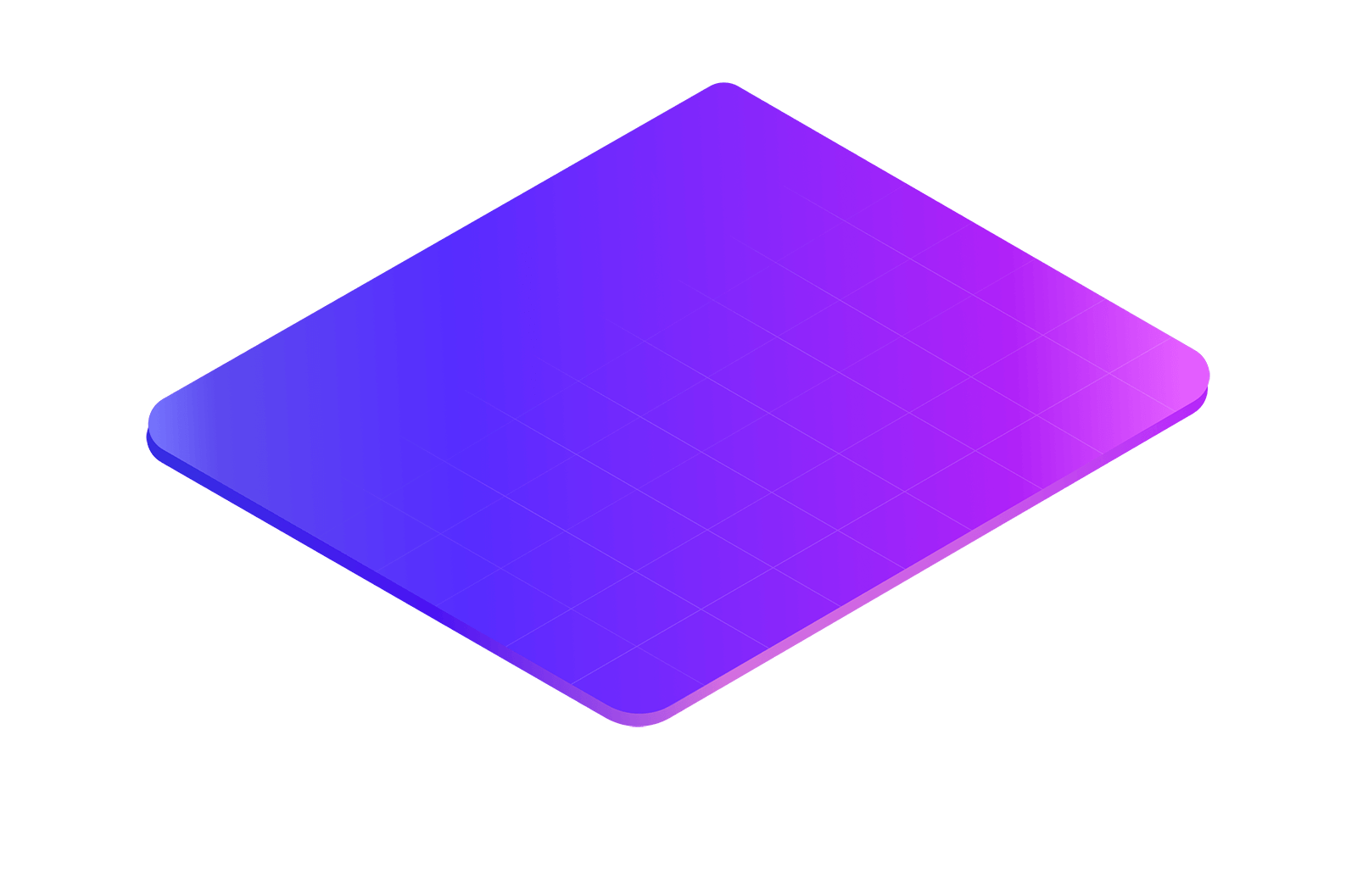
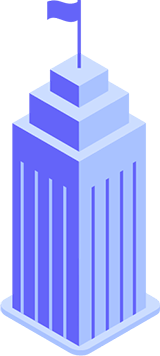
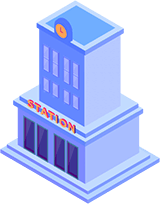
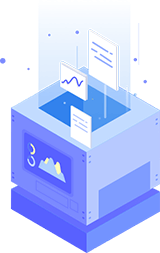
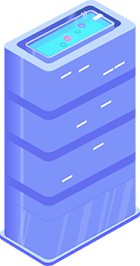
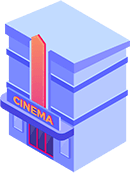
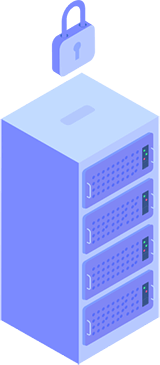
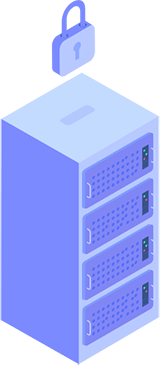
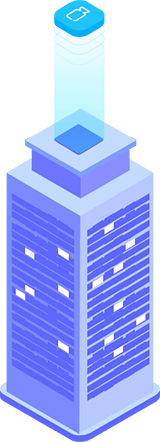
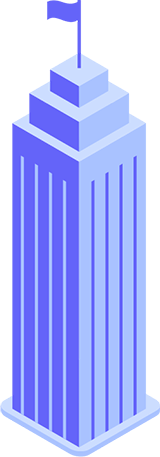
Play your role
LORDLESS is a fantasy game where you rule your Tavern.
Recruit bounty hunters, send them on quests, and reap the rewards. You can also play a bounty hunter to complete the quests and get the rewards.
Host The host posts a quest to reach the desired goal. In the world of LORDLESS, the Quest host is the publisher of all quests and the provider of all quest rewards.
Tavern master The owner of a tavern is the Tavern master. Once you become a master, you get the right to buy and sell the tavern, as well as take the cut of rewards.
Bounty hunter Bounty hunters can take the quests posted by the host in different taverns. When they complete the quest, the bounty hunter will reap a corresponding reward.
Trading on OpenSea
OpenSea is the world’s largest digital marketplace for crypto collectibles. You can buy, sell, and discover exclusive digital assets.
#800
Shanghai Circus World
121.446536, 31.2797366
0x35d4**09710
Max AP
84
Level
53
Hunters
#1800
Shanghai Art Museum
121.490278, 31.1864101
0x35d4**09607
Max AP
71
Level
50
Hunters
#0
Oriental Pearl Tower
121.495376, 31.2416800
0x35d4**091,121
Max AP
131
Level
496
Hunters
#101
Jing'an Temple
12144.0712, 31.2253722
0x35d4**091,044
Max AP
122
Level
148
Hunters
#100
Bund 27, The House of Roosevelt
121.485552, 31.2423914
0x35d4**09949
Max AP
111
Level
107
Hunters
#801
Xujiahui Saint Ignatius Cathedral
121.431471, 31.1930143
0x35d4**09684
Max AP
80
Level
48
Hunters
#1801
Hengshan Moller Villa Hotel Shanghai
121.451688, 31.2248880
0x1a08**ed581
Max AP
68
Level
47
Hunters
#802
City God Temple of Shanghai
121.488054, 31.2277205
0x35d4**09616
Max AP
73
Level
34
Hunters
Smart contract
LORDLESS is built entirely on Ethereum. All smart contracts are interrelated to build an overall decentralized system.
pragma solidity ^0.4.24;
import "../lib/SafeMath.sol";
import "./ITavern.sol";
contract TavernBase is ITavern {
using SafeMath for *;
struct Tavern {
uint256 initAt; // The time of tavern init
int longitude; // The longitude of tavern
int latitude; // The latitude of tavern
uint8 popularity; // The popularity of tavern
uint256 activeness; // The activeness of tavern
}
uint8 public constant decimals = 16; // longitude latitude decimals
mapping(uint256 => Tavern) internal tokenTaverns;
function _tavern(uint256 _tokenId) internal view returns (uint256, int, int, uint8, uint256) {
Tavern storage tavern = tokenTaverns[_tokenId];
return (
tavern.initAt,
tavern.longitude,
tavern.latitude,
tavern.popularity,
tavern.activeness
);
}
function _isBuilt(uint256 _tokenId) internal view returns (bool){
Tavern storage tavern = tokenTaverns[_tokenId];
return (tavern.initAt > 0);
}
function _build(
uint256 _tokenId,
int _longitude,
int _latitude,
uint8 _popularity
) internal {
// Check whether tokenid has been initialized
require(!_isBuilt(_tokenId));
require(_isLongitude(_longitude));
require(_isLatitude(_latitude));
require(_popularity != 0);
uint256 time = block.timestamp;
Tavern memory tavern = Tavern(
time, _longitude, _latitude, _popularity, uint256(0)
);
tokenTaverns[_tokenId] = tavern;
emit Build(time, _tokenId, _longitude, _latitude, _popularity);
}
function _batchBuild(
uint256[] _tokenIds,
int[] _longitudes,
int[] _latitudes,
uint8[] _popularitys
) internal {
uint256 i = 0;
while (i < _tokenIds.length) {
_build(
_tokenIds[i],
_longitudes[i],
_latitudes[i],
_popularitys[i]
);
i += 1;
}
}
function _activenessUpgrade(uint256 _tokenId, uint256 _deltaActiveness) internal {
require(_isBuilt(_tokenId));
Tavern storage tavern = tokenTaverns[_tokenId];
uint256 oActiveness = tavern.activeness;
uint256 newActiveness = tavern.activeness.add(_deltaActiveness);
tavern.activeness = newActiveness;
tokenTaverns[_tokenId] = tavern;
emit ActivenessUpgrade(_tokenId, oActiveness, newActiveness);
}
function _batchActivenessUpgrade(uint256[] _tokenIds, uint256[] __deltaActiveness) internal {
uint256 i = 0;
while (i < _tokenIds.length) {
_activenessUpgrade(_tokenIds[i], __deltaActiveness[i]);
i += 1;
}
}
function _popularitySetting(uint256 _tokenId, uint8 _popularity) internal {
require(_isBuilt(_tokenId));
uint8 oPopularity = tokenTaverns[_tokenId].popularity;
tokenTaverns[_tokenId].popularity = _popularity;
emit PopularitySetting(_tokenId, oPopularity, _popularity);
}
function _batchPopularitySetting(uint256[] _tokenIds, uint8[] _popularitys) internal {
uint256 i = 0;
while (i < _tokenIds.length) {
_popularitySetting(_tokenIds[i], _popularitys[i]);
i += 1;
}
}
function _isLongitude (
int _param
) internal pure returns (bool){
return(
_param <= 180 * int(10 ** uint256(decimals))&&
_param >= -180 * int(10 ** uint256(decimals))
);
}
function _isLatitude (
int _param
) internal pure returns (bool){
return(
_param <= 90 * int(10 ** uint256(decimals))&&
_param >= -90 * int(10 ** uint256(decimals))
);
}
}